The topic for today was "software security theory". Four different domains were presented:
- Type systems
- Static analysis
- Proof carrying code
- Program monitor
Type safety has to do with data structures in memory. In order to keep memory separated between processes, virtual memory is used. Still, there are potential back doors using shared memory regions and inter process calls using functionality of the operating system. Different programming languages operate at different abstraction levels. Machine code has no restrictions other than what the hardware allows. Compiled languages tend to have different sorts of checking and protections against misuse. The goal is to avoid errors, and also to be able to spot errors by inspecting the code. Features of higher level programming languages is that memory handling are automated, and that permissions are checked during run-time, at the cost of less performance (resources are being used for administration). Data is structured in types: boolean, integers, characters, floats, strings (arrays), objects etc, and a compiler can use type checking in static (compile time) and dynamic (tun time) in order to verify correct operation. Both in order to avoid mistakes (turning a percentage into an integer for example) and for avoiding access to uninitialized areas. Having automated initialization (and clearing) of memory after use protects from possible confidentiality and spoofing attacks. We looked at some examples of different languages and how they deal with type checking on adding an integer 5 to a string "37":
Javascript
<html>
<body>
<script type="text/javascript">
var x = 5;
var y = "37";
var z = x + y;
alert(typeof(z) + " of value " + z)
</script>
</body>
</html>
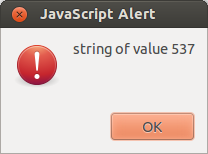
Java script would create a concatenation, resulting in the string: "537"
Visual Basic
var x := 5;
var y := "37";
var z := x + y;
If performed in Visual Basic (and probably PHP too), the output will be 42.0 (a float).
Python 2
x = 5
y = "37"
z = x + y
print("%s of value %s") % (type(z), z)

If done in Python we get an error
C
#include<stdio.h>
int main()
{
int x = 5;
printf("%p \n", &x);
char y[] = "37";
printf("%p \n", y);
char* z = x + y;
printf("%p \n", z);
return 1;
}
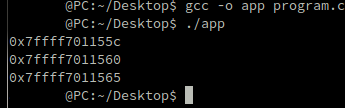
In C using the code bellow you would get the reference in memory + 5 of the "y" variable
The summary of this is that unless you know the language very well, there are many possible outcomes one could think of, and thus makes it difficult proving behavior. In other words "lack of predictability". In strict typed languages you would expect errors, and in loose typed you often find the language being quite forgiving.
Static analysis is about doing checks at compile time like semantics, type checking, looking for uninitialized or not used code blocks, forbidden functions and calls etc. When based on heuristics we get problem with false positives and negatives.
Proof carrying code is as far as I understand not about proving the code is secure (bug free) but has to do with proving the origin of the code. Lets say an update from Microsoft for a Windows version. The patch is signed so that we can rely on it being issued from Microsoft and at the same time verify it has not been modified since release. Still it won't stop any bad code introduced at the code base (hacking) and by mistake. It's very hard to do right as the biggest problem is during development. Managed stores like the Apple store is an example of trying to do this. They will compile the source themselves and while doing it check for permissions and do any static analysis they can. This is enforced with certificates and signed as "claimed not malicious".
Program monitoring is trying to look after a running program. It can be enforces from the outside with firewalls and IDS'es, it can be performed online (same machine) with anti virus software, it can be performed by the operating system or a high level run time compiler (Sand-boxing). The main problem for online checking comes to: "Are you able to avoid smart hackers from just disabling your monitoring?"...
Only one presentation were held today, and it was about "Language Based Security".
- Minimal trust
- Least privilege
- Control flow, memory/stack/type safety
Static analyses checks variables type
Dynamic analysis checks values type
Java combines them both...